NpOptiX - base, headless raytracer
Ray-tracing and compute loop
Calculations in PlotOptiX are performed in threads parallel to the thread where you created plotoptix.NpOptiX
object. This design allows building interactive applications where heavy calulations are not blocking UI. There are two
threads: one used for the ray tracing work done by OptiX engine (GPU work) and the second thread where any user calculations,
e.g. scene updates, can be performed (usually using CPU).
Callbacks are provided to synchrionize user code and OptiX tasks. The full workflow is illustrated in figures below, however it is best explained with the example code. Note, code in calbacks should follow recommendations indicated in the Fig.1. and in the Callbacks section. The basic rules are:
Access results stored in the output buffers only when they are frozen (
on_launch_finished
,on_rt_accum_done
) or useon_rt_accum_done
to synchronize your code with the end of ray tracing; otherwise buffers may be still empty or contain undefined data.Put lenghty calculations in
on_scene_compute
so they can run in parallel to ray tracing. Heavy computations in other callbacks will degrade overall performance.Do the scene and configuration updates in
on_rt_completed
. Note that most updates will reset the accumulation frame counter and the whole ray tracing loop will be restarted, which will also clear the output buffers.
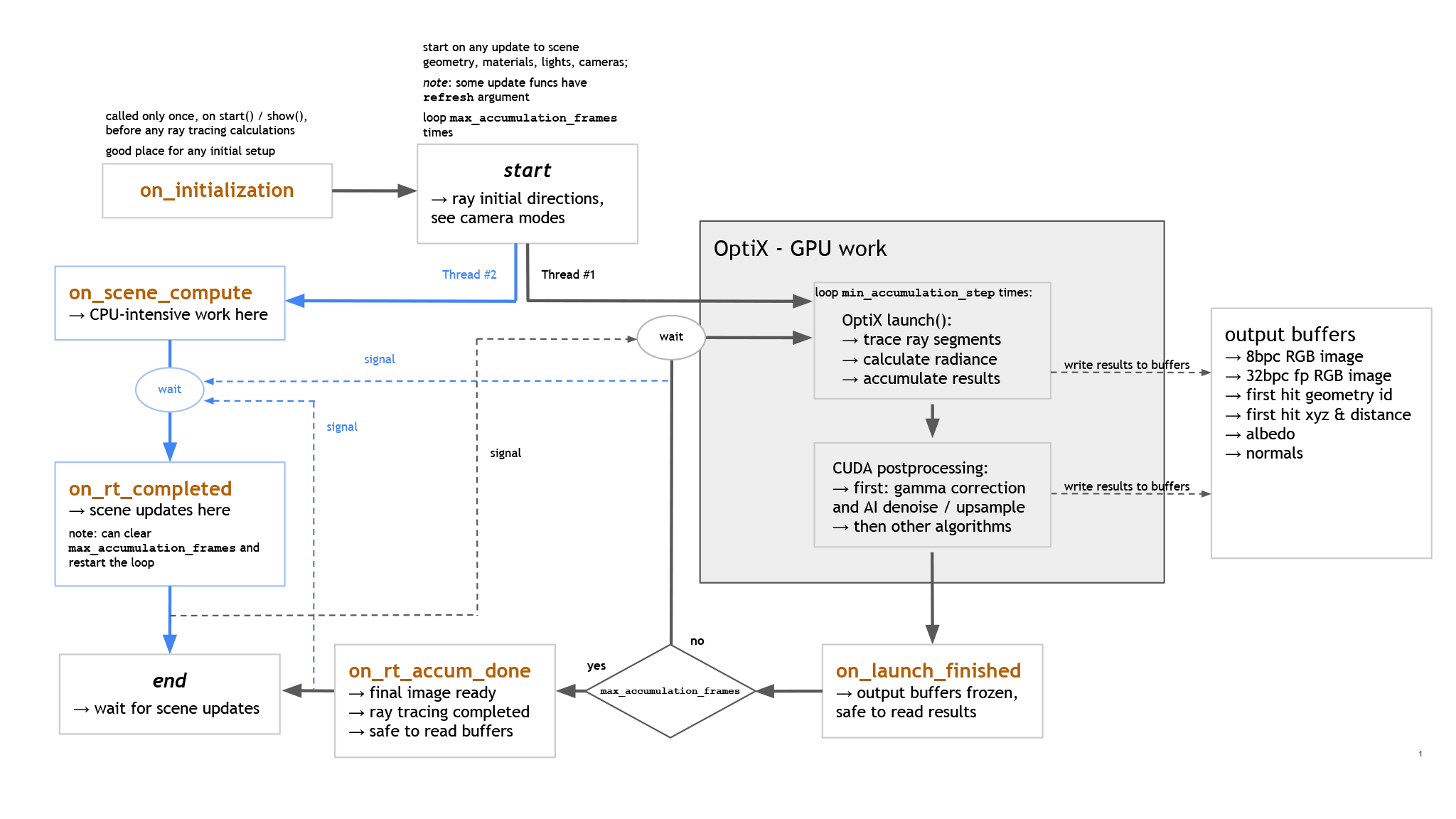
Fig. 1. PlotOptiX computations flow. Details of the OptiX task are ommited for clarity (i.e. scene compilation and multi-gpu management).
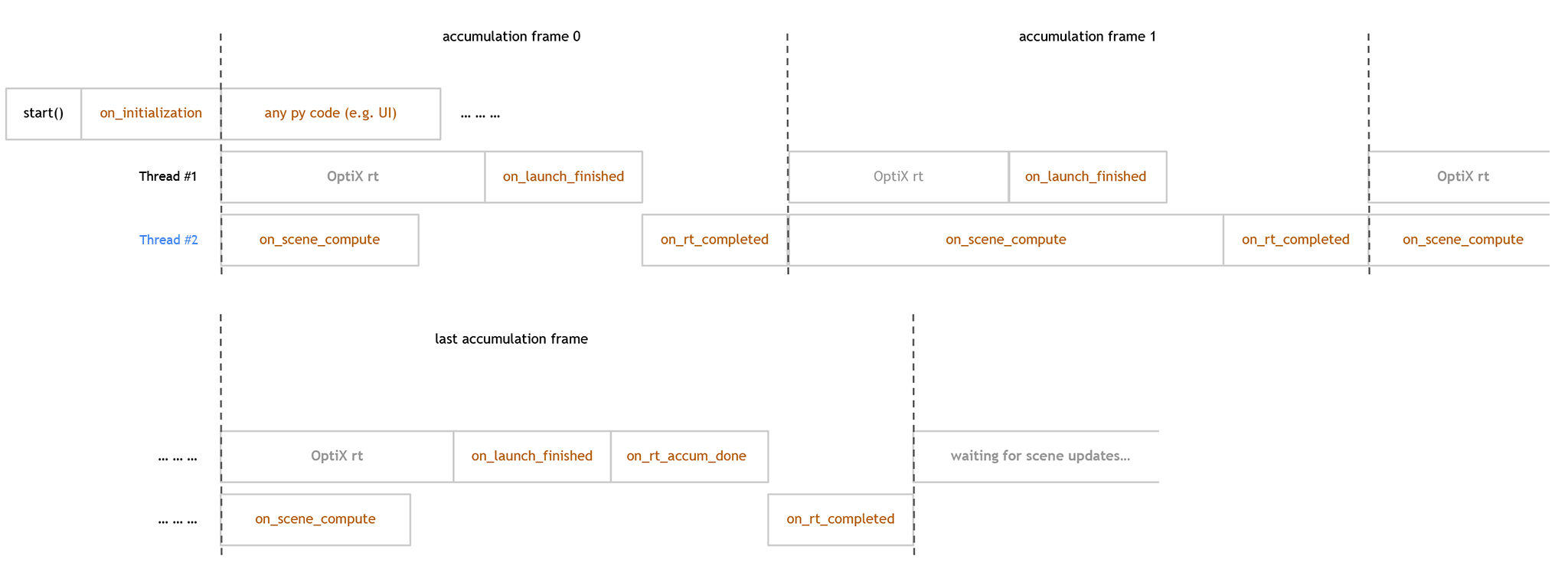
Fig. 2. Timeline of tasks and callbacks. Note, ray tracing work and on_scene_compute
are launched in parallel threads and on_rt_completed
is executed when all other task finished.
API reference
- class plotoptix.NpOptiX(*args, **kwargs)[source]
No-UI raytracer, output to numpy array only.
Base, headless interface to the RnD.SharpOptiX raytracing engine. Provides infrastructure for running the raytracing and compute threads and exposes their callbacks to the user. Outputs raytraced image to numpy array.
In derived UI classes, implement in overriden methods:
start and run UI event loop in:
plotoptix.NpOptiX._run_event_loop()
raise UI close event in:
plotoptix.NpOptiX.close()
update image in UI in:
plotoptix.NpOptiX._launch_finished_callback()
optionally apply UI edits in:
plotoptix.NpOptiX._scene_rt_starting_callback()
- Parameters
src (string or dict, optional) – Scene description, file name or dictionary. Empty scene is prepared if the default
None
value is used.on_initialization (callable or list, optional) – Callable or list of callables to execute upon starting the raytracing thread. These callbacks are executed on the main thread.
on_scene_compute (callable or list, optional) – Callable or list of callables to execute upon starting the new frame. Callbacks are executed in a thread parallel to the raytracing.
on_rt_completed (callable or list, optional) – Callable or list of callables to execute when the frame raytracing is completed (execution may be paused with pause_compute() method). Callbacks are executed in a thread parallel to the raytracing.
on_launch_finished (callable or list, optional) – Callable or list of callables to execute when the frame raytracing is completed. These callbacks are executed on the raytracing thread.
on_rt_accum_done (callable or list, optional) – Callable or list of callables to execute when the last accumulation frame is finished. These callbacks are executed on the raytracing thread.
width (int, optional) – Pixel width of the raytracing output. Default value is 16.
height (int, optional) – Pixel height of the raytracing output. Default value is 16.
start_now (bool, optional) – Start raytracing thread immediately. If set to
False
, then user should callstart()
method. Default isFalse
.devices (list, optional) – List of selected devices, with the primary device at index 0. Empty list is default, resulting with all compatible devices selected for processing.
log_level (int or string, optional) – Log output level. Default is
WARN
.
API Reference
- Start, configure, get output
NpOptiX.start()
NpOptiX.close()
NpOptiX.get_scene()
NpOptiX.set_scene()
NpOptiX.load_scene()
NpOptiX.save_scene()
NpOptiX.save_image()
NpOptiX.get_rt_output()
NpOptiX._img_rgba
NpOptiX._raw_rgba
NpOptiX._hit_pos
NpOptiX._geo_id
NpOptiX._albedo
NpOptiX._normal
NpOptiX._run_event_loop()
NpOptiX.run()
NpOptiX.get_gpu_architecture()
NpOptiX.enable_cupy()
NpOptiX.enable_torch()
- Scene configuration
- Raytracer configuration
- Postprocessing 2D
- Encoder configuration
- Geometry
- Cameras
- Lights
- Materials
- Callbacks